Operators That Multiply and Divide
These are familiar to anyone who has even used a spreadsheet program.
The Multiplicative Operators
- The asterisk
*
is used for multiplication
- The forward slash
/
is used for division
- The percent sign
%
is the modulo operator. (What's this, you ask? Don't worry; we'll go over it in good detail.)
Multiplying
- You can multiply numbers and number variables.
- When you multiply a number and a string the string will be converted to a number by JavaScript.
- Even when you multiply a string by a string JavaScript will convert the strings to numbers, if it can.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 | // Multiplying numbers
let numberOne;
let numberTwo;
let numberStringThree;
let multiplyResult;
numberOne = 25;
numberTwo = 100;
numberStringThree = "20";
multiplyResult = numberOne * numberTwo;
// Multiplying numbers directly
document.write("You can just multiply numbers right here: ");
document.write(75 * 34);
document.write("<br />");
// Multiplying number variables
document.write("The result of multiplying " + numberOne + " times "
+ numberTwo + " is: " + multiplyResult + "<br />");
// Multiplying numbers and strings?
multiplyResult = numberOne * numberStringThree;
document.write("The result of multiplying " + numberOne + " times \""
+ numberStringThree + "\" is: "
+ multiplyResult + " <-- Huh? <br /><br />");
document.write("What happens when you multiply two strings? <br />");
document.write('"200" * "34" = ');
document.write("200" * "34");
|
Dividing
- You can divide numbers and variables.
- When you divide a number and a string the string will be converted to a number by JavaScript.
- Even when you divide a string by a string JavaScript will convert the strings to numbers, if it can.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 | // Dividing numbers
let numberFour;
let numberFive;
let numberStringSix;
let divisionResult;
numberFour = 250;
numberFive = 7;
numberStringSix = "25";
divisionResult = numberFour / numberFive;
// Dividing numbers directly
document.write("You can just divide numbers right here: ");
document.write(75/34);
document.write("<br />");
// Dividing number variables
document.write("The result of " + numberFour + " divided by "
+ numberFive + " is: " + divisionResult + "<br />");
// Dividing numbers and strings?
divisionResult = numberFour / numberStringSix;
document.write("The result of " + numberFour + " divided by \""
+ numberStringSix + "\" is: "
+ divisionResult + " <-- Hmm...<br /><br />");
document.write("What happens when you divide two strings? <br />");
document.write('"200" / "34" = ');
document.write("200" / "34");
|
Modulo Operator
The modulo operator is very simple. Remember back in elementary school when you learned to do division manually? They called it "long division" in my era. The modulo operator just gives you the remainder when you divide two numbers.
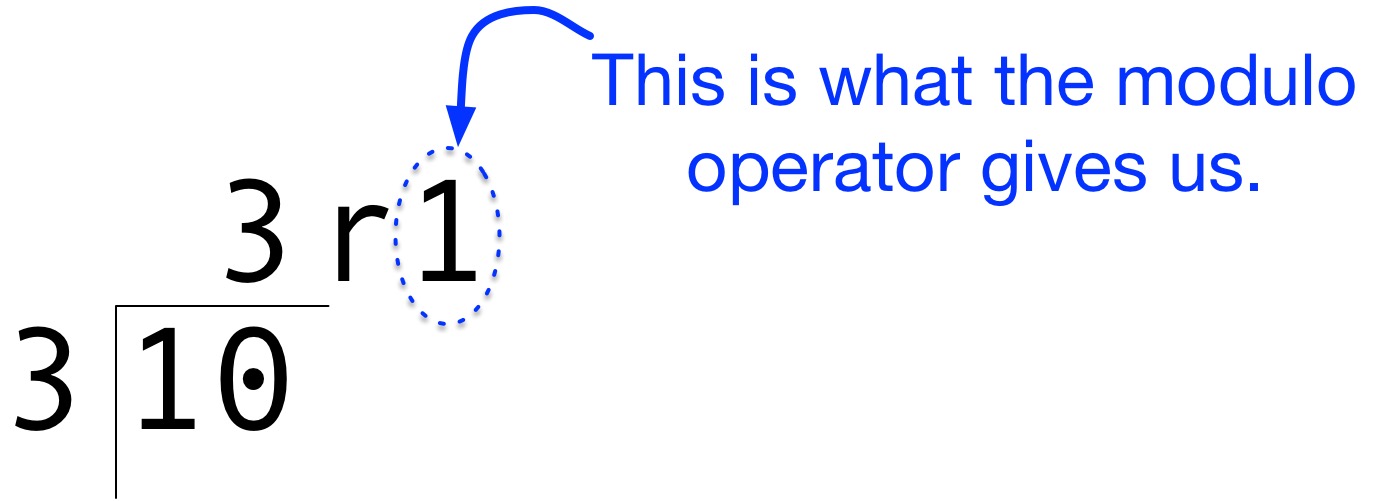
Here's how we code it in JavaScript
| // Modulo operator
let result;
let result = 10 % 3;
document.write("Ten modulo Three is: " + result);
|
And here are more examples
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | // Modulo examples
let fortyFive;
let six;
let four;
let moduloResult;
fortyFive = 45;
six = 6;
four = 4;
moduloResult = fortyFive % six;
document.write("The remainder of " + fortyFive + " divided by "
+ six + " is: " + moduloResult + "<br />");
moduloResult = fortyFive % four;
document.write("The remainder of " + fortyFive + " divided by "
+ four + " is: " + moduloResult);
|
Read more
Related Reading
Pages 187 - 188
Hands on work
This lab is all about multiplying and dividing. And you thought you wouldn't have to do that anymore in school!
Labs
- Lab05: Multiplicative Operators
- unit02/labs/lab-05-multiplicativeOperators.html
Exercises
- Exercise05: Exercise for lab05
- unit02/exercises/exercise-05.html