Nested if Statements, Linear and Non-Linear
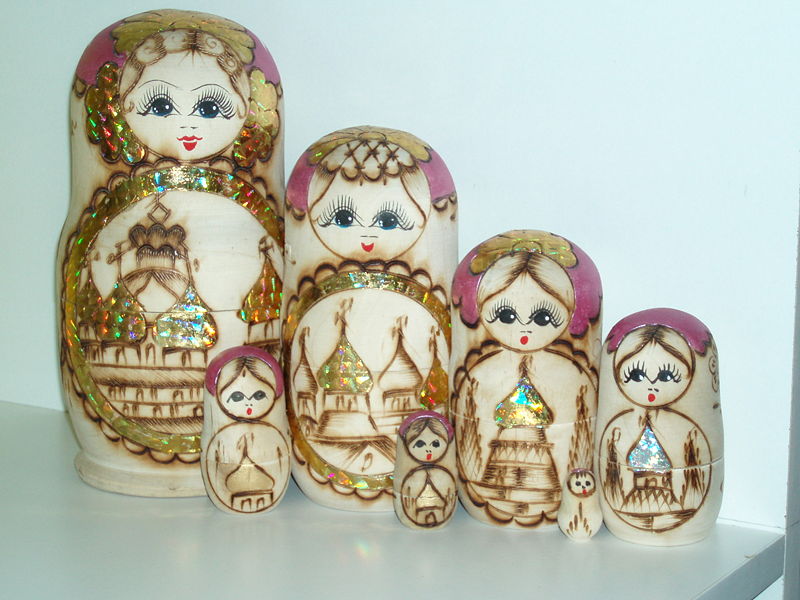
Nested if/else
Statements
What if you want to answer more than one question in a series? Or you want to test a variable for one of many options? We frequently have situations like this in programs. Here's some code snippets that illustrates a common problem
| // Nested if statements
if (choice1 === 'A') {
if (choice2 === 2) {
if (choice3 === "Blue") {
//Do a really important task!
}
}
}
|
This is the same as using multiple logical AND (&&
) expressions. But, with one important difference, we can use else
statements, too.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19 | // Nested if statements with else statements
if (choice1 === 'A') {
if (choice2 === 2) {
if (choice3 === 'X') {
if (choice4 === "Blue") {
// All true
} else {
// 'A', 2, 'X' are true but not "Blue"
}
} else {
// 'A', 2 are true but not 'X'
}
} else {
// 'A' is true but not 2
}
} else {
// 'A' is not true
}
|
How's this look? A bit complicated? It can get worse...
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62 | // Deeply nested if statements
if (true) {
if (true) {
if (true) {
if (true) {
//do something
} else {
//do something
}
} else {
if (true) {
//do something
} else {
//do something
}
}
} else {
if (true) {
if (true) {
//do something
} else {
//do something
}
} else {
if (true) {
//do something
} else {
//do something
}
}
}
} else {
if (true) {
if (true) {
if (true) {
//do something
} else {
//do something
}
} else {
if (true) {
//do something
} else {
//do something
}
}
} else {
if (true) {
if (true) {
//do something
} else {
//do something
}
} else {
if (true) {
//do something
} else {
//do something
}
}
}
}
|
But, this kind of coding is not that typical. Over time programmers have figured out that this is very fragile and you never really know if it works right. We now have lots of ways to avoid this type of monster.
We'll start with some basic patterns of nesting our logic
.
Linear Nested if
Statements
The linear nested if statement allows us to do many things like testing one variable for many options, and range testing. It uses a new key concept in programming: else if
.
Here's how it's used.
Very frequently, we will also have an else
statement at the end.
| // if/else if
if (<boolean expression>) {
// code
} else if (<boolean expression>) {
// code
}
|
Demo
- Demo: Linear Nested ifs
- unit03/demos/demo-linear-nested-ifs.html
Here's a larger example. We have a choice variable and the user is asked to make a selection from a list. Doing this with deeply nested if/else statements is ugly! So most languages have better ways. This is usually called the "if, else if, else if, else" pattern among programmers. It looks like this.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24 | function nestedIfs1() {
// One variable, many choices
let choice;
choice = "G";
if (choice === "A") {
document.write("The choice was A");
} else if (choice === "B") {
document.write("The choice was B");
} else if (choice === "C") {
document.write("The choice was C");
} else if (choice === "D") {
document.write("The choice was D");
} else if (choice === "E") {
document.write("The choice was E");
} else if (choice === "F") {
document.write("The choice was F");
} else if (choice === "G") {
document.write("The choice was G");
} else {
document.write("The choice was none of the above");
}
}
|
This is a very useful and commonly used structure. The above code replaces what's below and does exactly the same work. Which would you rather do?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30 | // Long version. Yuck!
if (choice === "A") {
document.write("The choice was A");
} else {
if (choice === "B") {
document.write("The choice was B");
} else {
if (choice === "C") {
document.write("The choice was C");
} else {
if (choice === "D") {
document.write("The choice was D");
} else {
if (choice === "E") {
document.write("The choice was E");
} else {
if (choice === "F") {
document.write("The choice was F");
} else {
if (choice === "G") {
document.write("The choice was G");
} else {
document.write("The choice was none");
}
}
}
}
}
}
}
|
Testing Different Conditions With if/else
structures.
** Click me to run nestedIfs2()
**
Note: Refresh the page to return to the course content
Sometimes we just have to nest if/else
statements as it won't work any other way. We try to limit it and make it concise.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30 | function nestedIfs2() {
// Nested ifs
let state;
let county;
let city;
state = prompt("What State do you live in?");
if (state === "WI") {
county = prompt("What county do you live in?");
if (county === "Dane") {
city = prompt("What city in Dane county do you live in?");
if (city === "Madison") {
document.write("Madison is a nice place");
} else {
document.write("There are other cities in Dane County?");
}
} else {
city = prompt("What city do you live in?");
if (city === "Milwaukee") {
document.write("That's too big for me.");
} else {
document.write("I don't know much about that city.");
}
}
} else {
document.write("Goodbye!");
}
}
|
If we limit the complexity we can manage.
Range Testing
We frequently need to test to see if a value is within a range. The technique to solve this combines nested if/else
with multiple booleans.
Pick a number between 1 and 10
** Click me to run nestedIfs3()
**
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23 | function nestedIfs3() {
// Range Testing
const LOWER_LIMIT = 1;
const UPPER_LIMIT = 10;
let numberEntry;
let number;
numberEntry = prompt("Pick a number between 1 and 10");
if (isNumeric(numberEntry)) {
number = Number(numberEntry);
} else {
alert("Entered value for number is not numeric");
return;
}
if (number >= LOWER_LIMIT && number <= UPPER_LIMIT) {
document.write("You got it right: " + number);
} else {
document.write("Your entry was out of range.");
}
}
|
Using this technique we can pick from multiple ranges.
** Click me to run nestedIfs4()
**
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38 | function nestedIfs4() {
// Multiple ranges
const LOWER_LIMIT = 0;
const RANGE_1 = 10;
const RANGE_2 = 20;
const RANGE_3 = 30;
const RANGE_4 = 40;
const RANGE_5 = 50;
let numberEntry;
let number;
numberEntry = prompt("Pick a number between 1 and 50");
if (isNumeric(numberEntry)) {
number = Number(numberEntry);
} else {
alert("Entered value for number is not numeric.");
return;
}
if (number < LOWER_LIMIT) {
document.write("That number is negative, I don't do negative.");
} else if (number >= LOWER_LIMIT && number <= RANGE_1) {
document.write(number + " is between " + LOWER_LIMIT + " and " + RANGE_1);
} else if (number > RANGE_1 && number <= RANGE_2) {
document.write(number + " is between " + RANGE_1 + " and " + RANGE_2);
} else if (number > RANGE_2 && number <= RANGE_3) {
document.write(number + " is between " + RANGE_2 + "and " + RANGE_3);
} else if (number > RANGE_3 && number <= RANGE_4) {
document.write(number + " is between " + RANGE_3 + " and " + RANGE_4);
} else if (number > RANGE_4 && number <= RANGE_5) {
document.write(number + " is between " + RANGE_4 + " and " + RANGE_5);
} else {
document.write(number + " is bigger than " + RANGE_5 + ".");
}
}
|
Demo
- Demo: Tax Code
- unit03/demos/demo-tax-code.html
Anyone who has never made a mistake has never tried anything new. -Albert Einstein
Labs
- Lab07: Nested ifs
- unit03/labs/lab-07-nestedIfs.html
Exercises
- Exercise for lab07
- unit03/exercises/exercise-07.html