Simple if/else Statement
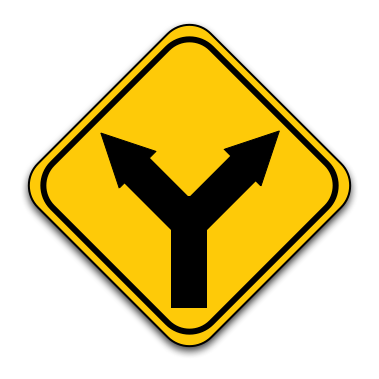
The if/else
Statement
- Now we can do something if the if the condition is
false
.
- If the boolean expressions is
true
then the code after the if
is run. If it is false
then the code after the else
is run.
| // Simple if/else
if (some condition) {
// do something
} else {
// do something else
}
|
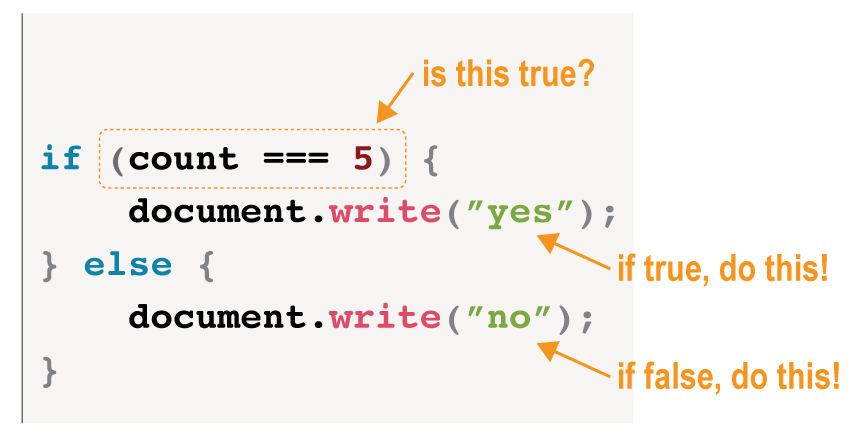
New Coding Standard
Compound Statements
Notice the spacing before and after the else
. Review the coding standards for compound if statements.
Examples
Branching false
| function simpleIfElse1() {
let count;
count = 4;
if (count === 5) {
document.write("yes");
} else {
document.write("no");
}
}
|
Branching true
| function simpleIfElse2() {
let price;
price = 14.99;
if (price < 15.00) {
document.write("I'll buy it!");
} else {
document.write("That's too much for me.");
}
}
|
Where it gets interesting is when we won't know which way it will go until the program is running.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55 | function simpleIfElse3() {
// Constant Declarations
const SMALL_PURCHASE_LIMIT = 24.99;
const SMALL_DISCOUNT = 0.05;
const LARGE_DISCOUNT = 0.1;
// Variable Declarations
let total;
let subTotal;
let priceOne;
let priceOneEntry;
let priceTwo;
let priceTwoEntry;
// Get user input
priceOneEntry = prompt("Enter 1st price.");
priceTwoEntry = prompt("Enter 2nd price.");
// Check first entry for numeric value
if (isNumeric(priceOneEntry)) {
priceOne = Number(priceOneEntry);
} else {
alert("Entered value for first price was not numeric.");
}
// Check second entry for numeric value
if (isNumeric(priceTwoEntry)) {
priceTwo = Number(priceTwoEntry);
} else {
alert("Entered value for second price was not numeric");
}
// Calculate subtotal
subTotal = priceOne + priceTwo;
// Calculate total
if (subTotal > SMALL_PURCHASE_LIMIT) {
total = subTotal - (subTotal * LARGE_DISCOUNT);
} else {
total = subTotal - (subTotal * SMALL_DISCOUNT);
}
// Display output differently depending on the discount
document.write("Your total with a ");
if (subTotal > SMALL_PURCHASE_LIMIT) {
document.write("BIG ");
} else {
document.write("small ");
}
document.write("discount on a purchase of $" + subTotal
+ " is $" + total);
}
|
Validating strings
Previously, we covered how to check to see if a user entered a numeric value, but what about strings? There are a lot of things we can do to validate a string, but for now, we are just going to make sure the user entered a value. We can do this with the .length
property. Recall, that the .length
property can only be used on string - which is what we always get from a prompt()
. If the length of an input is zero, we can assume the user entered nothing!
Checking strings
| let userName;
userName = prompt("Please enter your name.");
if (userName.length === 0) {
alert("You did not enter anything, please try again.");
}
|
Demo
- Demo: Divide Safely
- unit03/demos/demo-divide-safely.html
Read more
Related Reading
Pages 39 - 46
Hands on Work
Labs
- Lab05: Simple if/else
- unit03/labs/lab-05-simpleIfElse.html
Exercises
- Exercise for lab04
- unit03/exercises/exercise-04.html